Hi guys,
I’ve got some problems with coding a addProductsToCart function on a standard button.
The dataset is filtered with the field that I want on a dynamic page.
I’m looking for “automating” the function so that I don’t have to write all the _Id for each products that I want to add to cart …
Do you think it’s possible ?
This is the code I’ve written :
export function addBundle_click(event, $w) {
const productId = $w( ‘#dynamicDataset’ ).getItems( 1 , 5 )._id;
$w( ‘#shoppingCartIcon1’ ).addProductsToCart(productId, 1 )
}
In this example, there are 5 filtered Products in the database but some other page have 20…
I’ve done this for a single product and it works very well with the .getCurrentItem() function…but it’s only for one _id…
This is the code I use for single one product :
export function addToCartBtn_click(event, $w) {
const productId = $w( ‘#dynamicDataset’ ).getCurrentItem()._id;
$w( “#shoppingCartIcon1” ).addToCart(productId, 1 ,);
}
Thank you for your help,
Ludovic
@ludoviccorpsconscien The addProductsToCart function expects an array with specific field names that the code below creates by looping through the getItems result:
export function addBundle_click(event, $w) {
$w('#dynamicDataset').getItems(1,5)
.then((result) => {
console.log(result);
let products = [], item = {};
result.items.forEach((product) => {
item = {"productID": product._id,"quantity": 1}
products.push(item);
})
console.log(products);
$w("#shoppingCartIcon1").addProductsToCart(products)
.then( () => {
console.log("addProductsToCart was successful");
} );
})
}
@tony-brunsman Thank you very much for your answer and the time that you spent coding 
I’ve tried the code and it nearly works 
It puts into cart 4 products (from 1 to 4) but not the 5th one.
I’ve tried to change the value between .getItems() but it seems not changing anything.
PS : There really are 5 filtered products in my database.
Thank you
export function addBundle_click(event, $w) {
$w('#dynamicDataset').getItems(1,5)
It’s 0-based, so it should be
$w('#dynamicDataset').getItems(0,5)
Oh okay…sorry I’m quite a newbie in coding ^^
That’s wonderful it works very well. THANK YOU @tony-brunsman
@tony-brunsman One last question please 
If I don’t filtered the database, can I use the quite same code to choose all the products having the same “Collection” name in the Collection field ?
And then create different buttons for each collection ?
In the picture bellow, the filtered collection name is “MINIMAL HOUSE” but there are 15 other names in my database.
Thank you
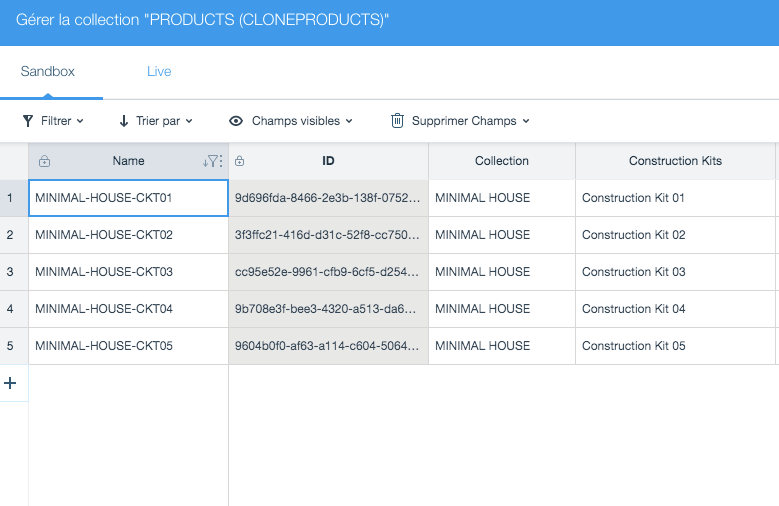
You could poll for how many records that are in the filtered dataset as follows:
let count = $w("#dynamicDataset").getTotalCount();
$w('#dynamicDataset').getItems(0,count)
.then ...
@tony-brunsman I mean something inspired on the code you’ve already given that loops the function addProductsToCart but this time according to the Collection field in the database :
Something like :
-
Go to database
-
Take only the products according to the field named “Collection”
-
Consider only the products having the Collection named “MINIMAL HOUSE” or “TRIP HOP”, or …
-
Then get their _id
-
Loop all their _id into addProductsToCart function
Thank you 
export function addBundle_click(event, $w) {
$w('#dynamicDataset').getItems(_Collection, All count)
.then((result) => {
console.log(result);
let products = [], item = {};
result.items.forEach((product) => {
item = {"productID": product._id,"quantity": 1}
products.push(item);
})
console.log(products);
$w("#shoppingCartIcon1").addProductsToCart(products)
.then( () => {
console.log("addProductsToCart was successful");
} );
})
}